The __init__ method is a key part of Python’s object-oriented programming (OOP). It’s used to initialize objects in Python and is often referred to as a constructor. In this article, we’ll break down what the __init__ method does, its syntax, and why it’s important in Python programming. Whether you’re new to Python or looking to deepen your OOP understanding, this article will guide you through the essentials of the __init__ method.
Introduction to Object-Oriented Programming in Python
Object-Oriented Programming (OOP) is a programming paradigm that organizes code around objects rather than functions and logic. In OOP, everything is treated as an object, which is an instance of a class. This approach offers several advantages:
- Modularity: OOP promotes modular code, making it easier to manage and organize large software projects.
- Reusability: Objects and classes can be reused across different parts of a program or even in different projects.
- Encapsulation: OOP allows data (attributes) and functions (methods) to be bundled together within an object, providing a way to control access and modification of data.
- Inheritance: OOP supports inheritance, allowing new classes to be derived from existing classes, promoting code reuse, and establishing a relationship between parent and child classes.
- Polymorphism: OOP facilitates polymorphism, enabling objects of different classes to be treated as objects of a common superclass.
Python, being a versatile and high-level programming language, fully supports OOP. At the heart of OOP in Python are:
- Classes: Blueprints for creating objects.
- Objects: Instances of classes, encapsulating data and behavior.
- Attributes: Variables within a class that hold data.
- Methods: Functions within a class that perform actions.
Defining Classes and Objects
In Python, defining a class is the first step to implementing Object-Oriented Programming (OOP) concepts. A class serves as a blueprint or template for creating objects. It encapsulates data for the object and methods to manipulate that data. Once a class is defined, you can create multiple objects or instances of that class, each with its own unique set of attributes and behaviors.
Key Components of a Class:
- Attributes: These are variables that hold data associated with the class and its objects. Attributes are defined within the class and are accessed using the dot notation with object names.
Methods: These are functions defined within the class that perform actions or operations on the data. Methods can be used to modify the attributes of an object or to perform specific tasks related to the class.
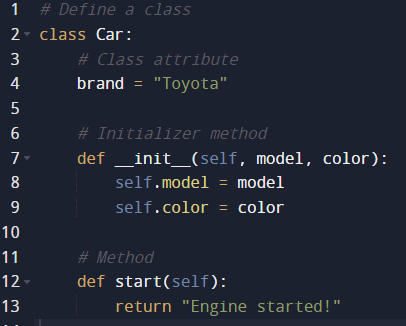
To create an object from the Car class:

Classes vs. Objects: A Primer
While the terms “class” and “object” are often used interchangeably, they have distinct meanings in OOP:
- Class: A blueprint or prototype that defines the variables (attributes) and methods (functions) common to all objects of a certain kind.
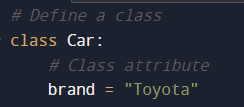
Object: An instance of a class. It’s a concrete entity based on the class, having its own set of attributes and methods.

Here’s a breakdown of the differences with examples:
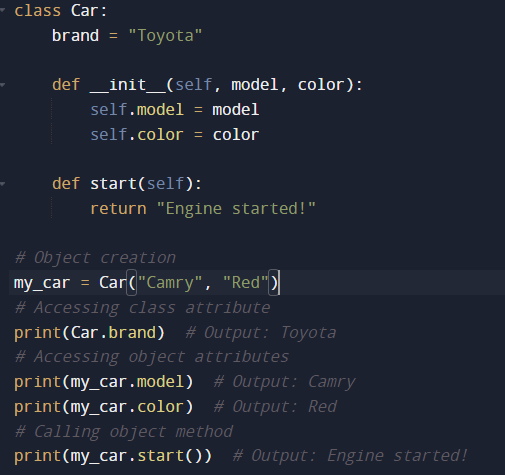
In this example:
- Car is a class with a class attribute brand.
- my_car is an object (instance) of the Car class with attributes model and color.
- We can access the class attribute using the class name (Car.brand) and object attributes using the object name (my_car.model, my_car.color).
The Role of the __init__ Method
The __init__ method is a special method in Python classes and plays a fundamental role in object initialization. It is often referred to as a constructor method because it is automatically called when an object is instantiated from a class. The primary purpose of the __init__ method is to initialize the object’s attributes.
Here’s a brief example to demonstrate the role of the __init__ method:
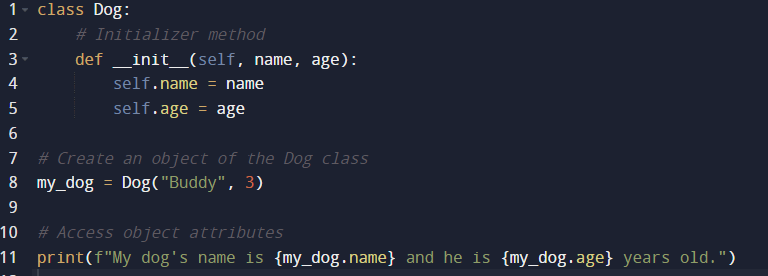
In this example:
- The __init__ method is defined with two parameters: name and age.
- When the Dog class is instantiated with my_dog = Dog(“Buddy”, 3), the __init__ method is automatically called to initialize the name and age attributes of the my_dog object.
How to Use the __init__ Method
To use the __init__ method, you define it within the class, specifying the attributes that you want to initialize. Inside the __init__ method, you use the self parameter to refer to the object being created and assign values to its attributes.
Code with the init method in python:
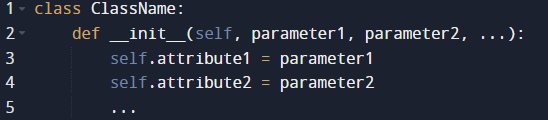
By utilizing the __init__ method, you can ensure that objects are initialized with the required attributes, making your code more organized and maintainable.
Practical Examples of __init__ in Use
The __init__ method is essential for initializing object attributes, and its practical application is widespread in Python programming. Here are a few practical examples to demonstrate the use of the __init__ method:
- Example 1: Employee Class
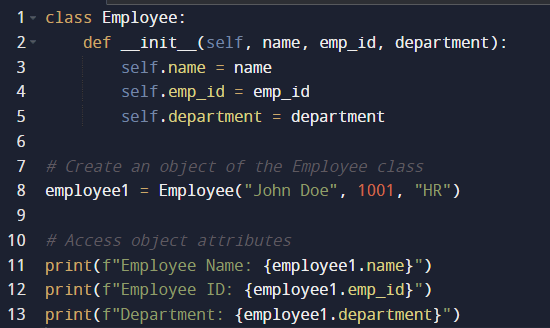
Output:
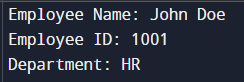
- Example 2: Book Class
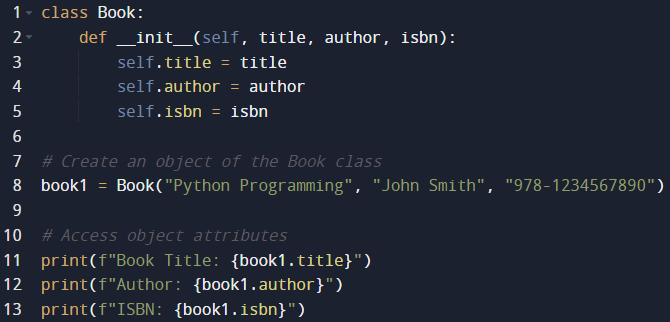
Output:
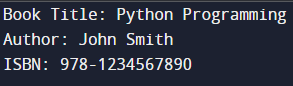
Note! In Python, the self parameter in the __init__ method refers to the instance of the class. It is a convention in Python to use self as the first parameter in instance methods to access and modify the object’s attributes within the class. While self is a convention and widely used, technically, you can use any other word as the first.
Default Constructors and Parameter Initialization
In Python, the __init__ method can have default parameter values, which can be useful for providing default attribute values when an object is instantiated without specifying certain parameters.
Let’s see an example:
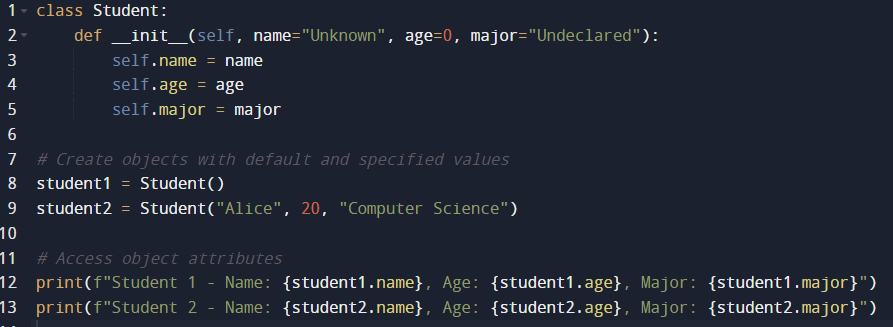
Output:

In this example:
- The Student class has a default constructor with default parameter values for name, age, and major.
- When student1 is instantiated without specifying any parameters, the default values are used.
- When student2 is instantiated with specific values, those values override the default values.
Using default constructors and parameter initialization in the __init__ method allows for flexibility and makes the class more user-friendly by providing default values when specific values are not provided during object instantiation.
Benefits of Using the __init__ Method:
- Consistency: The __init__ method ensures that all objects of a class are initialized with the required attributes, maintaining consistency across different instances of the class.
- Flexibility: By providing default parameter values in the __init__ method, you can create objects with default attributes while still allowing the flexibility to specify custom attribute values when needed.
- Readability: The __init__ method improves code readability by clearly defining and initializing the attributes of the class, making it easier to understand the structure and purpose of the class.
FAQ
- What is the __init__ method in Python?
The __init__ method in Python is a special method used within classes and plays a fundamental role in the object-oriented programming (OOP) paradigm. Referred to as the constructor in Python, __init__ is automatically invoked when an object is instantiated from a class, facilitating the initialization of the object’s attributes. Its primary purpose is to ensure that all objects created from the class are initialized with the required attributes, providing a consistent and structured approach to object initialization. The method uses the self parameter as a reference to the instance of the class, allowing for the access and modification of the object’s attributes within the class. The __init__ method can also have default parameter values, offering flexibility to provide default attribute values when an object is instantiated without specifying certain parameters.
- How does object-oriented programming apply in Python?
Object-oriented programming (OOP) is a programming paradigm that is fully supported and encouraged in Python, a versatile programming language. In Python, the fundamental building blocks of OOP are classes and objects. A class serves as a blueprint or template for creating objects, and defining the attributes (variables) and methods (functions) associated with the objects. An object is an instance of a class, and it encapsulates data in the form of attributes and code in the form of methods. One of the key principles of OOP is encapsulation, which involves bundling data (attributes) and methods (functions) that operate on the data into a single unit or class.