This article explores the problem of the default parameters in Java and seeks to shed light on the missing puzzle piece within the Java language’s features. We’ll look at the theory behind, and practice ways, in which Java developers create flexible coding approaches using default parameters.
The Concept of Default Parameters in Java
For a very long time, it has been widely known that Java is a flexible and stable language. However, one essential thing that has been missing is the creation of default parameters. Default parameters are used by developers to give default values for any function or method parameter lacking an argument. The problem is that while some languages such as Python and JavaScript have adopted this approach, java programmers had no choice but to use different tactics to attain a simulating functionality.
Simulating Default Parameters with Method Overloading
The common simple way in which Java developers simulate default parameters is through overloading. Overloading enables programmers to create more than one method with the same name but with different lists of parameters. The over-loading methods come with different combinations of parameter sets some of them contain the Java default values.
Let’s illustrate this with an example:
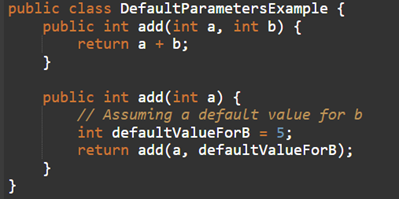
In this example, the add method is overloaded to accept two integers or just one. When only one integer is provided, the method assumes a default value for the second parameter (b).
While overloading offers flexibility and clarity, simulating defaults can challenge Java developers.
Challenges of Method Overloading in Java
- Readability Concerns. As the number of overloaded methods increases, readability may suffer. Developers must carefully choose Java default parameter values and ensure the code is easily understood.
- Maintenance Issues. Changing Java default parameter values or adding new parameters requires modifications to all overloaded methods. This can lead to maintenance challenges, especially in large codebases where tracking changes become complex.
- Limited Flexibility. Simulating default parameter in Java through overloading may not fully replicate the flexibility of true Java parameter default value. Developers must carefully design overloaded methods to accommodate various use cases, potentially leading to a more rigid API.
- Ambiguity Risks. If not designed thoughtfully, method overloading can introduce ambiguity, making it unclear which method should be called in certain situations. This can result in compilation errors or unexpected behavior.
Utilizing Nulls and Optional Parameters in Java
Now let’s also look into the smart application of nulls, and optional parameters as well. For example, a method may have several parameters, some of these can be set by default but also could be optional at the same time. Previously, one way to do this was to design several overloaded methods having distinct parameter collections. The other approach involves leveraging null values to signify the absence of an argument.
Default Parameters and Null Handling
In scenarios where default parameters are desired, developers can design methods to interpret null values as an indication to use Java default values. Let’s extend our previous example:
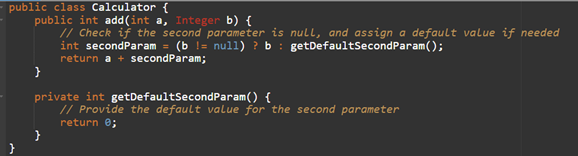
In this example, the add method checks whether the second parameter is null. If so, the method calls our private helper method, getDefaultSecondParam(), to retrieve the default value. This approach allows developers to achieve a semblance of default parameter in Java while handling null values intelligently.
Benefits and Considerations of Null Handling:
- Reduced Maintenance Complexity
- Improved Readability
- Adaptability to Optional Parameters
- Null Safety Considerations
The Builder Pattern: An Elegant Solution for Defaults
In Java design constructor patterns, the Builder Pattern stands out as an elegant simple solution for easily managing defaults and creating complex objects. This pattern provides a structured and fluent interface for constructing objects step by step, allowing developers to set default values and customize object creation. Let’s delve into the advantages of the Builder Pattern in Java.
Advantages of the Builder Pattern in Java
Let’s see an example:
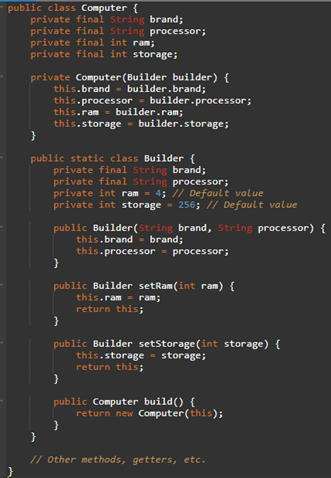
- Readable and Fluent API:
The code for creating a Computer object using the builder pattern is concise and easy to read.
- Default Values:
The builder pattern allows for default values to be set for optional parameters. In the code, default values for RAM and storage are clearly defined in the builder, simplifying the creation of objects.
- Flexible Object Creation:
The code showcases the flexibility of creating Computer objects step by step. Developers can set only the needed parameters.
- Immutable Objects:
The Computer class and its builder adhere to immutability principles. Once an object is constructed, its state cannot be changed.
- Reduced Constructor Overloading:
The builder pattern eliminates the need for multiple constructors with different parameter combinations. This results in cleaner code by centralizing the construction logic within the builder class.
- Encapsulation of Object Construction:
The builder pattern encapsulates the construction process within a separate class (Builder), promoting encapsulation and separation of concerns. This design decision makes the main class (Computer) more focused on its core functionality.
Leveraging var-args for Default Parameter Behavior
In Java, leveraging variable-length argument lists, often called var-args, can provide a flexible approach to achieving default parameter behavior. The syntax for var-args involves using three dots (…) followed by the parameter type.
Public int calculateSum(int… numbers)
One notable advantage is its flexibility in accepting arguments, including none, making the method adaptable to different use cases. This concise syntax simplifies method calls and allows developers to pass a dynamic number of parameters effortlessly. However, a significant disadvantage lies in the lack of type safety and the potential for runtime errors. Since var-args accept arguments of a specific type, developers need to ensure proper validation within the method body, leading to a risk of inadvertent type mismatches. Additionally, var-args can make the code less explicit, as the method signature alone may not convey the expected parameters, potentially hindering code readability and understanding.
The Role of Optional in Java Constructors
In Java, Optional class performs an important function in dealing with optional parameters in constructors. It elegantly indicates whether the value is there or not. Using option makes your code more readable, and adheres to good design practices while considering potential optional parameters for your constructor.
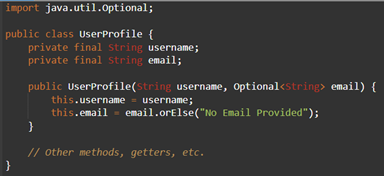
- Explicit Handling of Optionality:
The use of Optional makes it explicit that a parameter is optional. This enhances the readability of the code, indicating to developers that the value may or may not be present.
- Avoidance of Null Checks:
By using Optional, developers can avoid explicit null checks. The orElse method concisely specifies default method parameters when the optional parameter is absent.
- Encourages Defensive Programming:
Optional encourages a more defensive programming style. Developers are prompted to consider scenarios where values might be absent, leading to more robust and error-resistant code.
- Clear Intent in Constructors:
Constructors become more expressive when using Optional, as it conveys the intent that specific parameters are optional. This clarity in design contributes to better collaboration among developers.
- Consistent API Design:
Optional ensures consistency in API design when dealing with optional parameters. This consistency with default method parameters makes it easier for developers to understand and use various parts of the codebase.
FAQ
- How can Java default parameters be simulated since they are not natively supported?
Java does not natively support default parameters unlike such languages as Python or JavaScript, but developers can simulate them using several techniques. One common approach is overloading, where multiple method versions are created with different parameter sets. Another technique involves using null values for parameters that are meant to have default values. Optional parameters, introduced in Java 8 through the java.util.The Optional class provides a more elegant solution. By wrapping parameters in Optional, developers can choose whether to provide a value or use the default. The Builder Pattern is another helpful technique. Varargs (variable-length argument lists) provide simple way to simulate default parameters Java. By defining a method with varargs, developers can pass various Java default arguments.
- What are the limitations of using method overloading to simulate default parameters in Java?
The more overloaded methods there are, code redundancy and complexity will increase. In addition, parameter order should be carefully managed by the developers themselves to avoid confusion. Although overloading is an option, it may not be as clear as built-in support for default arguments.
- How does the builder pattern provide a solution for default parameters in Java?
The Builder Pattern offers a sophisticated solution by creating a separate builder class responsible for constructing objects with optional parameters. This approach improves code readability, maintains a clear API, and eliminates the need for numerous overloaded methods.